About this project
This project is all about how to create an automatic hand sanitizer using an Ultrasonic sensor and Arduino. You have already seen a lot many hand sanitizers based on IR sensors, and those hand sanitizers are really simple and easy to make. But those sanitizers are not efficient and accurate as hand sanitizers based on the ultrasonic sensors. The main problem with the IR sensor-based hand sanitizer is that you can’t place them under direct sunlight as the sunlight interferes with the IR sensor. Also, the maximum distance of the IR sensor for detecting the hand is less than 10cm but in an Ultrasonic sensor-based hand sanitizer you can set the distance to more than 10 cm.
Before moving to the project, let us first understand what an ultrasonic sensor is and how it works. The ultrasonic sensor is a type of sensor that is based on ultrasonic sound. It has one ultrasonic transmitter and one ultrasonic receiver. When you give an input signal to the sensor using a microcontroller the transmitter sends an ultrasonic wave, which then gets reflected back by the object present in front of the sensor and received by the ultrasonic receiver. The sensor then outputs the total time taken by sound to travel from the sensor and back to the sensor after reflecting from the object.
Pin diagram of the Ultrasonic sensor
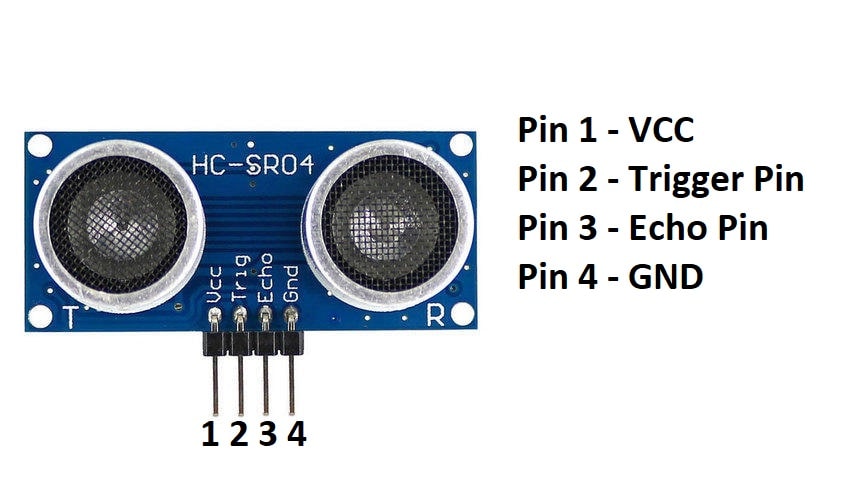
Working of the ultrasonic sensor
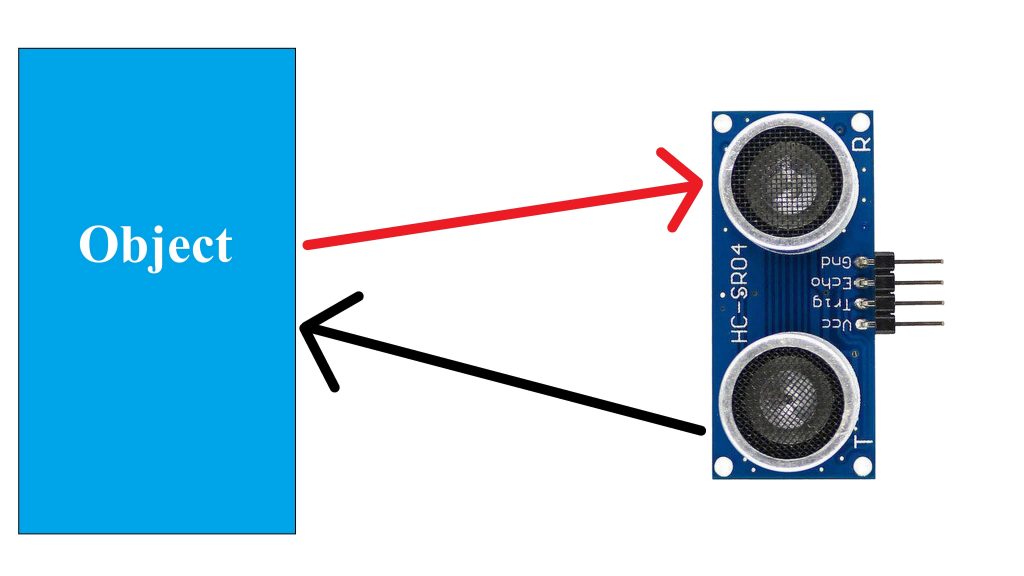
Working of the Automatic Hand Sanitizer
We will be using the ultrasonic sensor for the detection of the hand. The distance for detecting the hand can be easily set in the Arduino code based on your requirement. The Arduino will be repeatedly sending a signal to trigger the ultrasonic sensor and when your hand is present in front of the sensor then the sensor will output the total time taken by the sound to travel to and from the object. Then that signal is read by the Arduino. Based on that signal, we will be writing the code that when the sensor detects the hand it will turn on the hand sanitizer, and when the hand is not present in front of the sensor the hand sanitizer will be turned off. We will be using a DC water pump for this purpose.
Block diagram of Automatic Hand Sanitizer
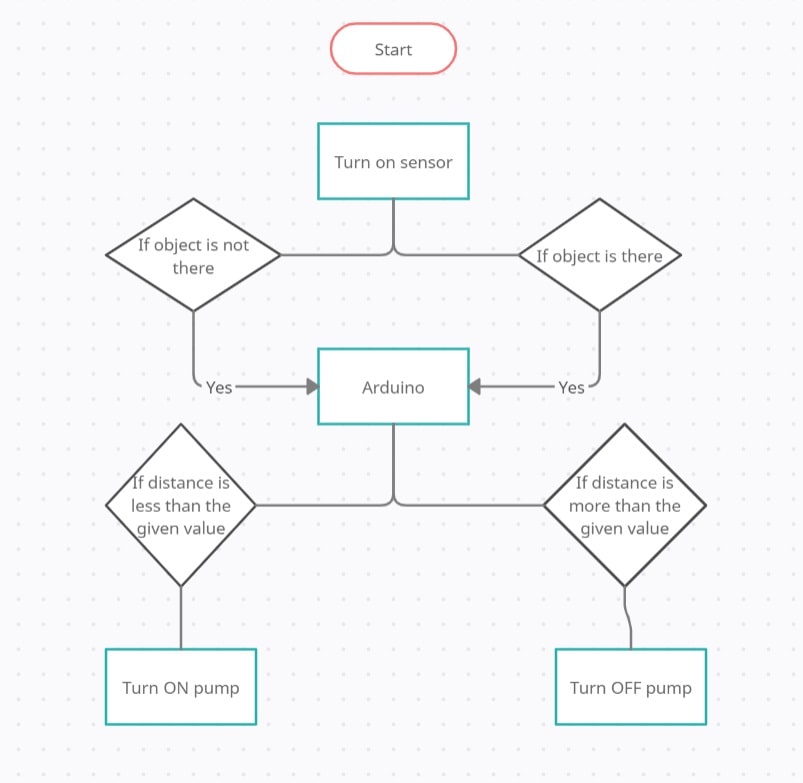
Circuit diagram of Automatic Hand Sanitizer
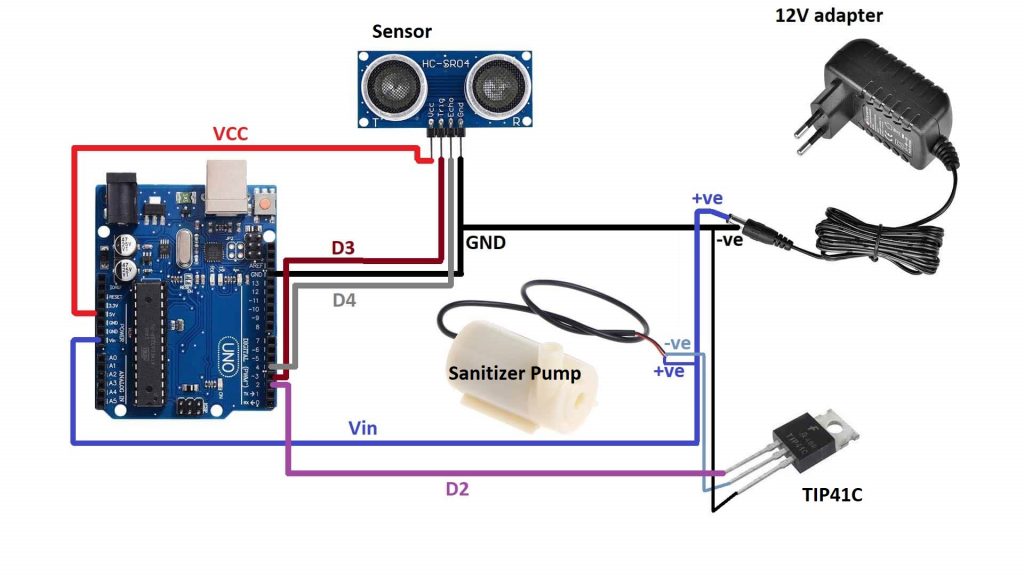
Arduino code for Automatic Hand Sanitizer
int pump=2;
int trig_Pin=3;
int echo_Pin=4;
long Time;
int distance;
void setup() {
Serial.begin(9600);
pinMode(trig_Pin,OUTPUT);
pinMode(echo_Pin,INPUT);
pinMode(pump,OUTPUT);
}
void loop() {
digitalWrite(trig_Pin,LOW);
delayMicroseconds(2);
digitalWrite(trig_Pin,HIGH);
delayMicroseconds(10);
digitalWrite(trig_Pin,LOW);
Time=pulseIn(echo_Pin,HIGH);
distance=Time*0.034/2;
Serial.print("Distance:");
Serial.println(distance);
if(distance<15)
{
digitalWrite(pump,HIGH);
}
else
{
digitalWrite(pump,LOW);
}
}
Working of code
int pump=2;
int trig_Pin=3;
int echo_Pin=4;
Create variables for storing the pin number where you have connected the signal pin of the water pump, trig pin and echo pin of the sensor.
long Time;
int distance;
Create one variable for storing the time from the sensor and one variable for storing the distance.
void setup() {
Serial.begin(9600);
pinMode(trig_Pin,OUTPUT);
pinMode(echo_Pin,INPUT);
pinMode(pump,OUTPUT);
}
Set the baud rate for the serial communication using serial.begin(). Then set the trig pin as output because Arduino will be using this pin to trigger the sensor. Then set the echo pin as input because Arduino will be using this pin to get the time data from the sensor.
void loop() {
digitalWrite(trig_Pin,LOW);
delayMicroseconds(2);
digitalWrite(trig_Pin,HIGH);
delayMicroseconds(10);
digitalWrite(trig_Pin,LOW);
In order to trigger the sensor, you have to send a logic HIGH on the trig pin for 10 microseconds. So, to do so first we will clear the trig pin by sensing logic LOW. then we will give a delay of 2us. Then we will send logic LOW on the trig pin and give a delay of 10us. And after that, we will send logic LOW to that pin.
Time=pulseIn(echo_Pin,HIGH);
distance=Time*0.034/2;
Then we will store the time data that is coming from the sensor using the pulseIN() function. After that, by using the formula speed=distance/ time we will calculate the distance of the object. We already know the speed of sound and time. So, you can easily calculate the distance. We have divided the value by 2 because the time that we will be getting from the sensor is the time taken by sound to travel from the sensor to object + time taken by sound to travel from object to sensor back. And we only need the one-way time that is why we have to divide it by 2. Here 0.034 is the speed of sound in cm/us because the time that we will be getting from the sensor is in microseconds.
Serial.print("Distance:");
Serial.println(distance);
The we will print the distance on the serial monitor using the print command.
if(distance<15)
{
digitalWrite(pump,HIGH);
}
We will check whether the distance of the hand is less than 15cm or not. You can set your distance here. If the distance is less than the given value the sanitizer pump will be turned on.
else
{
digitalWrite(pump,LOW);
}
If nothing is present in front of the sensor the sanitizer pump will be turned of.
Comments are closed.